In this article, we will focus on a useful feature provided by Sitecore XM / XM Cloud: Rendering Contents Resolvers. Multiple definitions are provided in the rendering definitions, which can be used to efficiently create components.
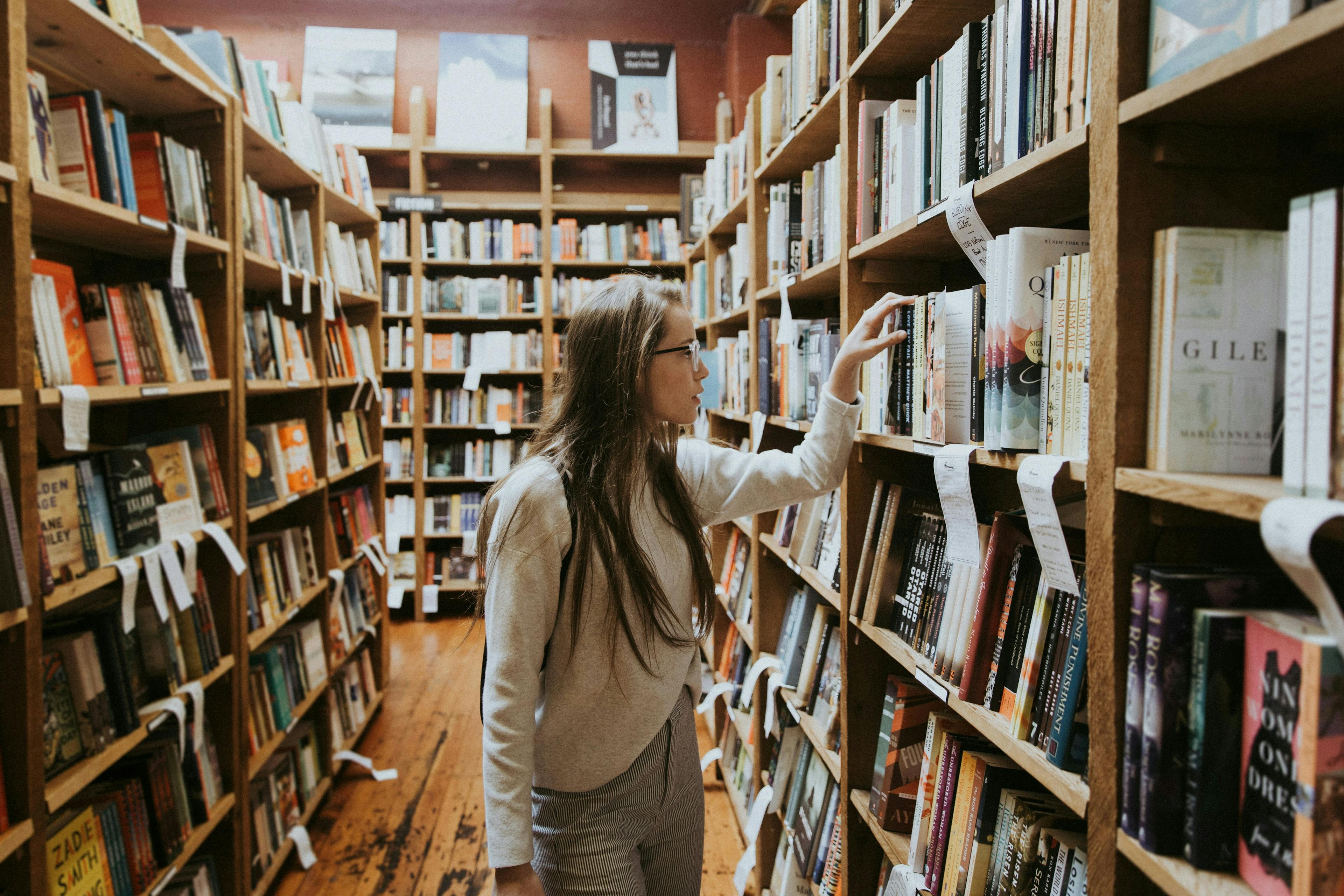
Retrieve data from layout services
The way the component retrieves data from Sitecore items is by using GraphQL, which was previously used for the breadcrumb list.
Alternatively, you can use the built-in Rendering Contents Resolver or incorporate a customized version. For more information on customization, please see the following page.
This setting is included in the Layout Service of the Rendering definition.

This time, we would like to confirm the operation of the Datasource Item Children Resolver, which uses child items as part of the built-in Rendering Contents Resolver.
Create a sample component
Prepare the code for the base component for validation. First, define heading and content (both in Single-Line Text) as templates.

Then, create the item as data using the template.

Finally, set up the code for this component as follows
import React from 'react';
import { ComponentParams, Field, RichText } from '@sitecore-jss/sitecore-jss-nextjs';
import { ComponentProps } from 'lib/component-props';
interface ContentBlockFields {
heading: Field<string>;
content: Field<string>;
}
interface TestComponentProps extends ComponentProps {
fields: ContentBlockFields;
params: ComponentParams;
}
export const Default = (props: TestComponentProps): JSX.Element => {
const id = props.params.RenderingIdentifier;
return (
<div className={`component ${props.params.styles}`} id={id ? id : undefined}>
<div className="component-content">
Heading - <RichText field={props.fields.heading} />
Content - <RichText field={props.fields.content} />
</div>
</div>
);
};
The sample now works as follows.

Datasource Item Children Resolver
This will be a Resolver that can retrieve data from items under the specified item. We will actually prepare a sample code. First, specify the Resolver for the test component as follows

The next step is to prepare the items. In this case, we have prepared three items.

The component code is then rewritten. We rewrote it all at once as follows.
import React from 'react';
import { ComponentParams, TextField } from '@sitecore-jss/sitecore-jss-nextjs';
import { ComponentProps } from 'lib/component-props';
interface PageItemFields {
displayName: string;
fields: {
Title: TextField;
};
}
interface TestComponentProps extends ComponentProps {
fields: {
items: PageItemFields[];
};
params: ComponentParams;
}
export const Default = (props: TestComponentProps): JSX.Element => {
const id = props.params.RenderingIdentifier;
return (
<div className={`component ${props.params.styles}`} id={id ? id : undefined}>
<div className="component-content">
<ul>
{props.fields.items.map((item, index) => (
<li key={index}>{item.fields.Title.value}</li>
))}
</ul>
</div>
</div>
);
};
This change lists the titles of the child items as follows.

When you add one, you will see that it has been added properly.

Summary
We have now seen how to render in a component using child items. In the next issue, we would like to create a component that uses this mechanism.