In the last issue, we proceeded to place and run the sample code. This time, we would like to check what kind of code is needed and in what form the data is held. What kind of code is needed to be written and in what form will the data be held?
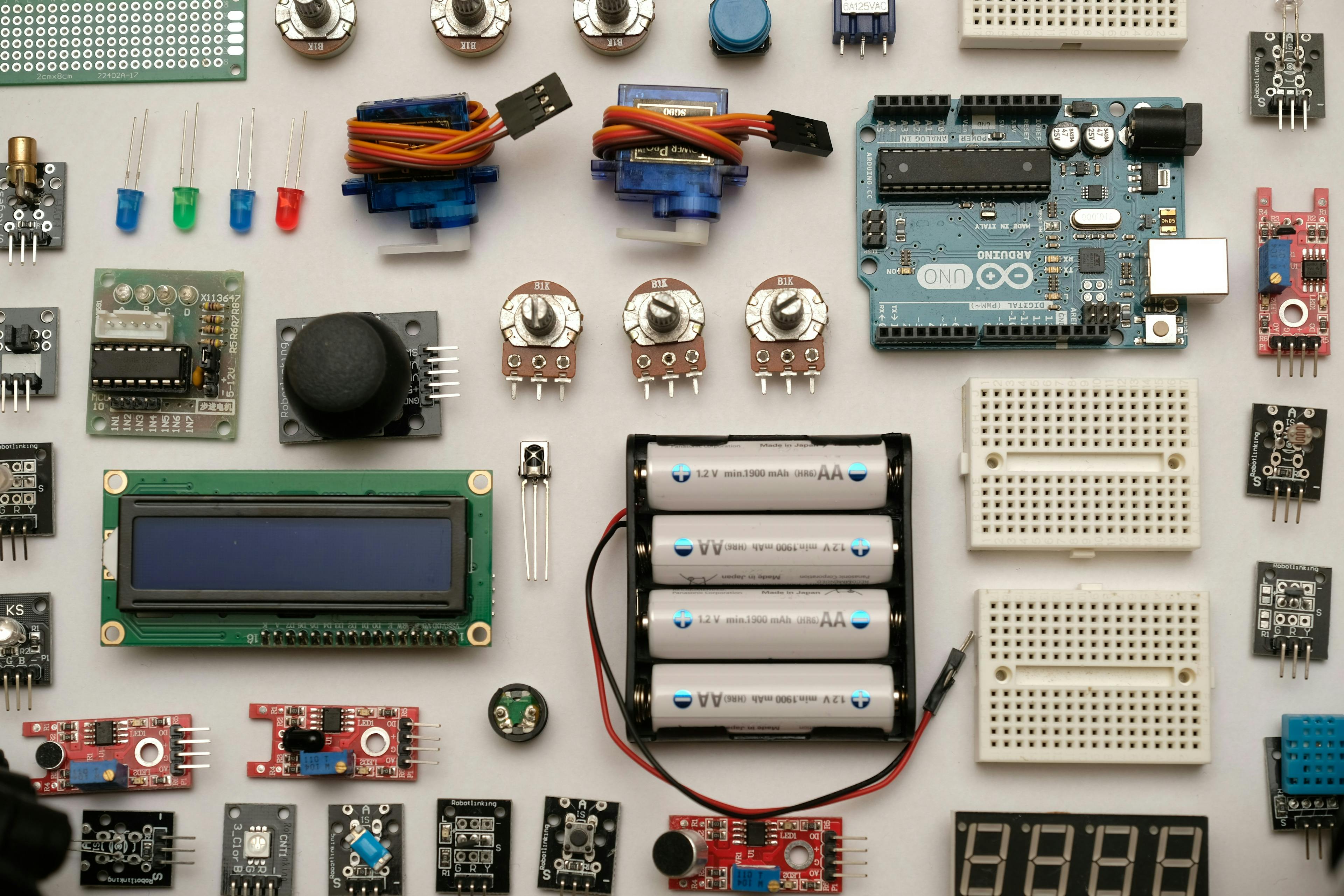
Review presentation
We would like to check the behavior of the sample component we created last time. First, if you check the presentation settings, you will see that the BYOC Wrapper control is in place.

Looking at the control data, the properties are set as follows

You can see that the actual configuration data is saved as Component Props.
We'll check the code.
We will then review the sample code. First of all, as for the layout part described in the component, it is as follows.
export default function ExampleClientsideComponent(props: {
firstName: string;
lastName: string;
telephone: string;
bold: boolean;
}) {
return (
<>
<h2>Hybrid</h2>
<dl style={props.bold ? { fontWeight: 'bold' } : {}}>
<dt>Description</dt>
<dd>Interactive UI with SEO-friendly fallback</dd>
<dt>Rendered on</dt>
<dd>{typeof window != 'undefined' ? 'Clientside' : 'Server'}</dd>
<dt>Data</dt>
<dd>
{props.firstName} {props.lastName} / {props.telephone}
</dd>
</dl>
</>
);
}
The above components are rendered using the following items.
- firstName: string;
- lastName: string;
- telephone: string;
- bold: boolean;
The codes listed below are definitions for the components.
FEAAS.registerComponent(ExampleClientsideComponent, {
name: 'hybrid',
title: 'Hybrid server/client',
description: 'Interactive UI with SEO-friendly fallback',
thumbnail: 'https://feaasstatic.blob.core.windows.net/assets/thumbnails/byoc.svg',
group: 'Examples',
required: ['firstName'],
properties: {
firstName: {
type: 'string',
title: 'First name',
},
lastName: {
type: 'string',
title: 'Last name',
},
telephone: {
type: 'number',
title: 'Telephone',
minLength: 10,
},
bold: {
type: 'boolean',
title: 'Show text in bold weight',
},
},
ui: {
firstName: {
'ui:autofocus': true,
'ui:emptyValue': '',
'ui:placeholder': 'Write your first name',
},
bold: {
'ui:widget': 'radio',
},
},
});
This section contains definitions regarding components.
- name - Component Name
- title - Component name as it appears in Pages
- group - Group name for displaying the component in Pages
- thumbnail - Thumbnail image of the component to be displayed in Pages
- required - required fields
- properties - With respect to the data held by the component
Now, at once, we have rewritten the code as follows. The information used will be the text, and the image to go with it.
import * as FEAAS from '@sitecore-feaas/clientside/react';
export default function ByoCSample(props: {
simpleText: string;
imageUrl: string;
imageText: string;
}) {
return (
<>
<h2>ByoC Sample</h2>
<dl>
<dt>Rendered on</dt>
<dd>{typeof window != 'undefined' ? 'Clientside' : 'Server'}</dd>
<dt>Data</dt>
<dd>
{props.simpleText} {props.imageUrl} / {props.imageText}
</dd>
</dl>
</>
);
}
FEAAS.registerComponent(ByoCSample, {
name: 'BYOCsample',
title: 'BYOC Sample',
description: 'Interactive UI with SEO-friendly fallback',
thumbnail: 'https://feaasstatic.blob.core.windows.net/assets/thumbnails/byoc.svg',
group: 'Tailwind Sample',
required: ['simpleText'],
properties: {
simpleText: {
type: 'string',
title: 'Component Text',
},
imageUrl: {
type: 'string',
title: 'Image URL',
},
imageText: {
type: 'string',
title: 'Alt Text',
},
},
ui: {
simpleText: {
'ui:autofocus': true,
'ui:emptyValue': '',
'ui:placeholder': 'simple name',
},
},
});
I just changed the items, but this is how I would like to run it this time. When actually placed, it will appear as follows.

We were able to confirm that we could place components and change their elements in Pages.
Generate plain components
In this case, we used the sample code to create the component, but it is possible to output the source code of the component with the command.
powershell jss scaffold Sample --byoc
The above command will generate the file src\components\Sample.tsx. The code is as follows
import React from 'react';
import * as FEAAS from '@sitecore-feaas/clientside/react';
interface SampleProps {
title: string;
columnsCount: number;
}
export const Sample = (props: SampleProps): JSX.Element => {
const columns: string[] = [];
for (let i = 0; i < props.columnsCount; i++) {
columns.push(`Component Column ${i + 1}`);
}
return (
<div className="container">
<h2>{props.title || 'BYOC Demo'}</h2>
<p>Sample Component</p>
<div className="row">
{columns.map((text, index) => (
<div key={index} className={`col-sm-${props.columnsCount}`}>
{text}
</div>
))}
</div>
</div>
);
};
FEAAS.External.registerComponent(Sample, {
name: 'Sample',
properties: {
title: {
type: 'string',
},
columnsCount: {
type: 'number',
},
},
});
Based on this code, we would create a component and register it.
Summary
In this case, we added a component to Sitecore using the "Bring own your component" mechanism. This is useful when using code from Figma, for example.