We will be covering Playwright on the blog for a while. We will be creating a simple example that addresses the need to use a screenshot of a page in a search result.
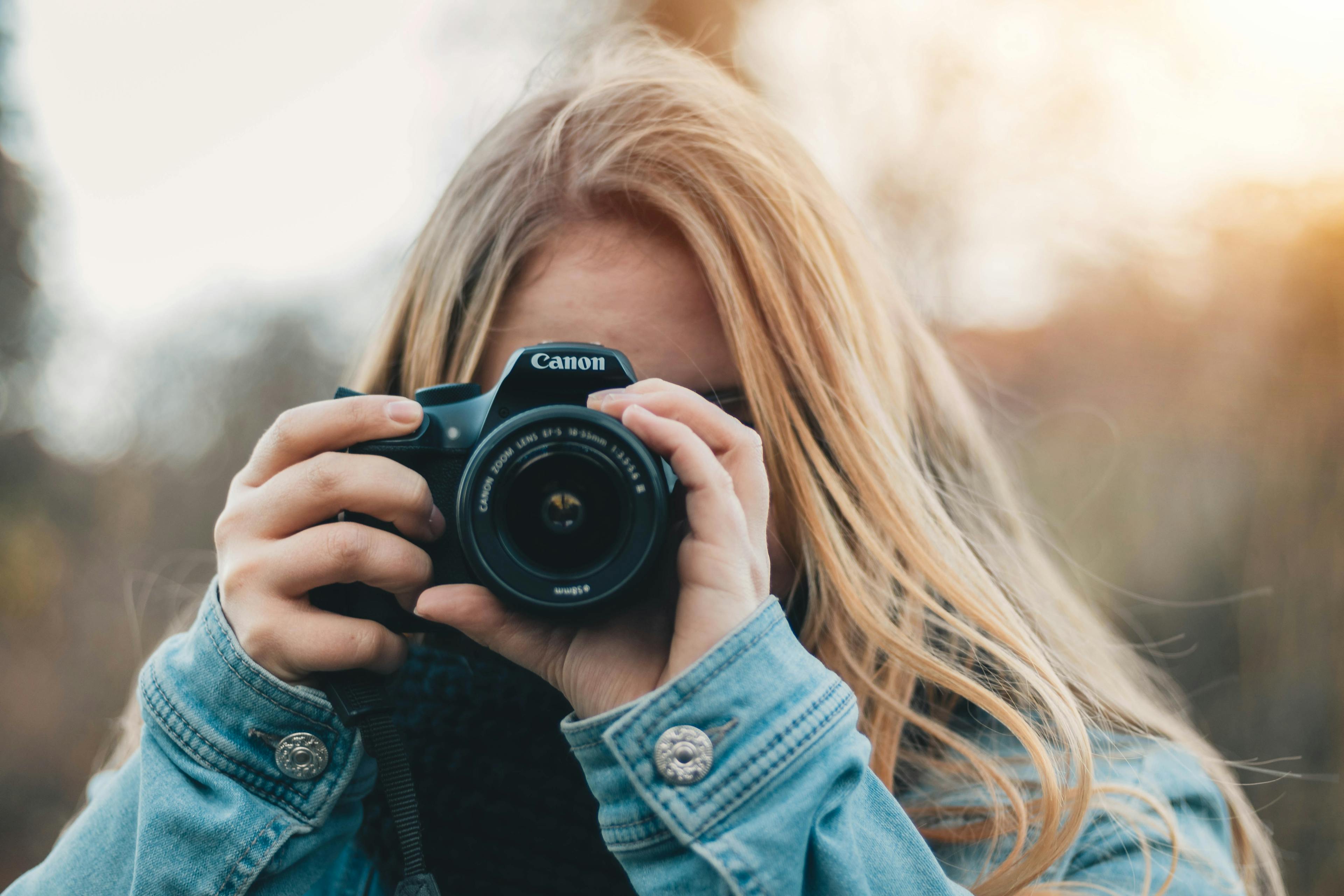
What is Playwright?
Two tools are often mentioned as being able to meet the need to automate testing using the browser's functionality.
We will proceed with this comparison, assuming Playwright, rather than the other way around. The main reasons are,
- Playwright has more browser support
- Playwright's development is handled by Microsoft and is frequently maintained.
Somewhere along the way, I have come to have a secure image regarding open source projects that are supported by Microsoft. For now.
Create a Typescript project
In this blog, I have introduced samples using Next.js and Vite, but this time, I will create a project using express to make it work like an API.
This time, we will work in a Windows environment where Node.js is available. First, create a project.
mkdir playwright
cd playwright
npm init -y

Add the necessary modules.
npm install express
npm install -D typescript @types/node @types/express
Next, create tsconfig.json. In this case, the following code was used to create the file.
{
"compilerOptions": {
"target": "ES6",
"module": "CommonJS",
"outDir": "./dist",
"rootDir": "./src",
"strict": true,
"esModuleInterop": true,
"skipLibCheck": true,
"forceConsistentCasingInFileNames": true
}
}
Since we are setting up a file under src, we first create the src/app.ts file as follows.
import express from "express";
import { hello } from "./routes/hello";
const app = express();
const port = 3000;
// API Test
app.get("/api/hello", hello);
// Handler when accessing the root path ('/')
app.get("/", (req, res) => {
res.send("Welcome to the Sitecore Search Utility");
});
app.listen(port, () => {
console.log(`Server is listening on port ${port}`);
});
The file that is being read in this file . /routes/hello file as src/routes/hello.ts.
// hello.ts
import { Request, Response } from "express";
export const hello = (req: Request, res: Response) => {
res.json({ message: "Hello World" });
};
Finally, rewrite the scripts section of package.json as follows
"scripts": {
"build": "tsc",
"dev": "node ./dist/app.js",
"start": "tsc && npm run dev",
"test": "echo \"Error: no test specified\" && exit 1"
},
Now you are ready to go. npm run build and you will see a compiled JavaScript file in the dist folder.

Then run the command. When you run the command npm run start, you will see the following.

If you actually access http://localhost:3000/ with a browser, you will see the following message

What happens when you access http://localhost:3000/api/hello that you have already created? You will see the following results

A simple express-based typescript sample is now available.
Summary
This article has been long, so I will end with an explanation of Playwright and an introduction to creating typescript samples. In the next article, we will build up to the point of taking a screenshot of a specified URL using Playwright.